invert binary tree iterative
Despite that implementing another way of traversing a tree adds a level of comprehension for key data structures such as stacks and queues as well as include the benefit of not having to worry. Can change only the values so that in-order traversal will generate a reversed list.
To invert the tree iteratively Perform the level order traversal using the queue.
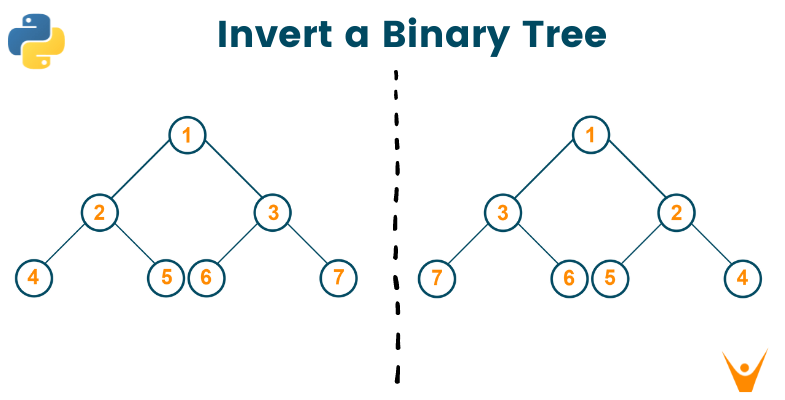
. This looks much better with binary search trees but that is not the original problem description. Given the root of a binary tree invert the tree and return its root. Swap the left and right pointers.
If node is None. The number of nodes in the tree is in the range 0 100-100. A recursive approach to insert a new node in a BST is already discussed in the post.
Our task is to create an inverted binary tree. Merge Two Binary Trees by doing Node Sum Recursive and Iterative Vertical Sum in a given Binary Tree Set 1. We traverse through all n nodes using recursion for on time complexity and we can have up to logn recursive calls on the stack at once where logn is the depth of the tree for ologn space complexity.
Classic examples are computing the size the height or the sum of values of the tree. Vertical Sum in Binary Tree Set 2 Space Optimized Find root of the tree where children id sum for every node is given. Use BFS to explore the tree and swap left and right child for every node.
The definition of a tree node is as follows. Python Server Side Programming Programming. Swapping the left and right child of every node in subtree recursively.
Invert A Binary Tree Recursive And Iterative Approach In Java The Crazy Programmer Leetcode Invert Binary Tree Solution Explained Java Youtube Share this post. 4 2 7 1 3 6 9. Inverting a binary tree means we have to interchange the left and right children of all non-leaf nodes.
Hint 1 Start by inverting the root node of the Binary Tree. So if the tree is like below. Performing an inversion would result in.
You can do so just as you did for the root. I use the function process node as a placeholder for whatever computation the problem calls for. In this tutorial I am going to discuss the iterative and recursive approaches to solve this problem.
Return None Swapping the children temp rootleft rootleft rootright rootright temp Recursion selfinvertrootleft selfinvertrootright return. Undoubtedly the iterative approach of traversing a binary tree traversal is a more verbose and complex solution when compared to its recursive counterpart. With BST this reversal produces a BST with a reversed comparison operator.
For converting a binary tree into its mirror tree we have to traverse a binary tree. How to invert a binary tree in cc. Store the root node in the queue and then keep on iterating the loop till the queue is not empty.
Show activity on this post. Def __init__ self val0 leftNone rightNone. Root 213 Output.
Node qpopleft if node. Return None temp nodeleft nodeleft noderight noderight nodeleft def levelOrder root. A new key is always inserted at the leaf node.
Find largest subtree sum in a tree. 1 2 5 3 4. In simple words Output is the mirror of the input tree.
Below are the three approaches to solve this problem. Hint 2 Once the first swap mentioned in Hint 1 is done you must invert the root nodes left child node and its right child node. 4 7 2 9 6 3 1.
Given the rootof a binary tree invert the tree and return its root. Converting recursive approach to iterative by using stack. 5 4 3 1 2.
In each iteration get the top node swap its left and right child and then add the left and right subtree back to the queue. Inverting this root node simply consists of swapping its left and right child nodes which can be done the same way as swapping two variables. So a tree that looks like.
You can invert a binary tree using recursive and iterative approaches. The example given on Leetcode shows an input tree. Inverting a binary tree can be thought of as taking the mirror-image of the input tree.
The inversion of a binary tree or the invert of a binary tree means to convert the tree into its Mirror image. To solve this we will use a recursive approach. Because rooted trees are recursive data structures algorithms on trees are most naturally expressed recursively.
I was working on a problem Invert Binary Tree in an iterative fashion. Inputroot 4271369Output4729631 Example 2. If the root is null then return.
90 of our engineers use the software you wrote Homebrew but you cant invert a binary tree on a whiteboard so f off - Max Howell Problem definition. Selfval val selfleft left selfright right from queue import Queue class Solution. Following is the code to invert a Binary Tree recursively.
If node is None. Suppose we have a binary tree. You can invert a binary tree using recursive and iterative approaches.
Using Iterative preorder traversal. Given a binary tree we have to invert the tree and print it. Space Complexity of Invert a Binary Tree.
You can invert a binary tree using recursive and iterative approaches. To implement binary tree we will define the conditions for new data to enter into our tree. Here are the three traversals.
Replace each node in binary tree with the sum of its inorder predecessor and successor. Binary Search Tree SET 1In this post an iterative approach to insert a node in BST is discussed. In this tutorial I have explained how to invert binary tree using iterative and recursive approachLeetCode June Challenge PlayList - httpswwwyoutubeco.
In simple terms it is a tree whose left children and right children of all non-leaf nodes are swapped. Function Nodeval thisval val. Iklan Tengah Artikel 1.
The inverted tree will be like. Root 4271369 Output. So if the tree is like below.
Given a binary tree like this. Tree is n. Root Output.
Definition for a binary tree node. Iklan Tengah Artikel 2. Reverse a binary tree in o1 in cc.
If root None. Insertion of a Key. We discuss different approaches to solve this problem along with their time and space complexities.
Newer Post Older Post Home. Return None q deque node root qappend node.
Top View And Bottom View Of A Binary Tree Data Structure Algorithm Youtube Binary Tree Data Structures Algorithm
Invert Binary Tree Iterative Recursive Approach
Invert Binary Tree Leetcode 226 Youtube
Invert A Binary Tree Python Code With Example Favtutor
Invert Reverse A Binary Tree 3 Methods
Python Inverting Binary Tree Recursive Stack Overflow
Numbering Of Nodes In A Fully Populated Binary Tree With L 3 Levels Download Scientific Diagram
Find Sum Of All Left Leaves In A Given Binary Tree Geeksforgeeks
Invert A Binary Tree Recursive And Iterative Solutions Learnersbucket
Invert Alternate Levels Of A Perfect Binary Tree Techie Delight
Find Least Common Ancestor Lca Of Binary Tree Learnersbucket
Invert A Binary Tree Interview Problem
Algodaily Invert A Binary Tree Description
Invert Binary Tree Iterative And Recursive Solution Techie Delight
Convert A Binary Tree Into Its Mirror Tree Geeksforgeeks
Invert A Binary Tree Bst Carl Paton There Are No Silly Questions
Inverting A Binary Tree In Python Theodore Yoong
Invert Reverse A Binary Tree 3 Methods
Invert A Binary Tree Recursive And Iterative Approach In Java The Crazy Programmer